HOW TO CREATE TODO APP BY CODEIGNITOR
To Get full tutorials Click Me
TODO APP
What is to do app ?
ToDo List App is a kind of app that generally used to maintain our day-to-day tasks or list everything that we have to do,
with the most important tasks at the top of the list, and the least
important tasks at the bottom. ... We can add more tasks at any time and
delete a task that is completed.
How to create Todo App by Codeignter Php framework
To create todo app in codeingnite follow the following step
1. Check your local machine contains Localserve[xampo,wampor lamp]
2. Download Codeingiter framework form www.codegniter.com
3. Extract Downloaded file and rename it to todoapp
4.Create database todo_db and configure it
5. Call your project on browser(localhost/todoapp).
Lets Start
Controller Function:
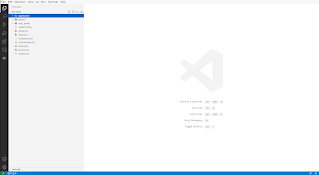
<?php
class Bf_Task extends CI_Controller{
public function __construct()
{
parent::__construct();
$this->load->model('Bf_Task_model','bft');
}
public function index()
{
$data['tasks']= $this->bft->getAllTask();
$this->load->view('task/list_task',$data);
}
public function Save_task(){
$result = $this->bft->Save_task();
if($result){
$this->session->set_flashdata('success_msg','Successfully Registered');
}
else{
$this->session->set_flashdata('error_msg','Failed to Registered');
}
redirect('Bf_Task/');
}
public function Update_task($id){
$result = $this->bft->Update_task($id);
if($result){
$this->session->set_flashdata('success_msg','Successfully Updated');
}
else{
$this->session->set_flashdata('error_msg','Failed to Update');
}
redirect('Bf_Task/');
}
public function Delete_Task($id){
$result = $this->bft->Delete_Task($id);
if($result){
$this->session->set_flashdata('success_msg','Successfully Deleted');
}
else{
$this->session->set_flashdata('error_msg','Failed to Delete');
}
redirect('Bf_Task/');
}
}
Model Function:
<?php
class Bf_Task_model extends CI_Model{
public function Save_task(){
$data=array(
'bf_task_name'=>$this->input->post('task'),
'status'=>0
);
$query = $this->db->insert('bf_task',$data);
if($query)
{
return true;
}
}
public function Update_task($id){
$data=array(
'bf_task_name'=>$this->input->post('task'),
'status'=>$this->input->post('status')
);
$this->db->where('bf_task_id',$id);
$query = $this->db->update('bf_task',$data);
if($query)
{
return true;
}
}
public function getAllTask(){
$this->db->select('*');
$this->db->from('bf_task');
$query = $this->db->get();
if($query){
return $query->result();
}
}
public function Delete_Task($id){
$this->db->where('bf_task_id',$id);
$query = $this->db->delete('bf_task');
if($query){
return true;
}
else{
return false;
}
}
}
View
<!DOCTYPE html>
<html>
<header>
<title>BF_Task</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity="sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin="anonymous"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin="anonymous"></script>
</header>
<body>
<div class="container">
<div class="row">
<div class="col col-md-12">
<div class="card">
<div class="card-header">
<h4>List of Tasks <a style="float:right;" href="#" class="btn btn-sm btn-success"><i class='fa fa-plus'></i> Add New Task</a></h4>
<form action="<?php echo base_url('Bf_Task/Save_task') ?>" method="post">
<input style="width: 90%; outline: none;border-radius: 3px; font-size: 30px; font-family: serif;" type="text" name="task" autofocus placeholder="Enter your task here">
<button class="btn btn-sm btn-info" style="font-size: 29px; margin-left: -10px;margin-top: -10px;" type="submit">+</button>
</form>
<table class="table table-borderless table-stripped">
<thead>
<tr>
<th>NO</th>
<th>Task</th>
<th>Duration</th>
<th>Status</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<?php $no = 0;
foreach ($tasks as $task) {
$no++; ?>
<tr>
<td><?php echo $no; ?></td>
<td><?php echo $task->bf_task_name ?></td>
<td><?php echo $task->created_at ?></td>
<td>
<?php
$status=$task->status;
if($status ==1 ){ ?>
<span class="text text-success">Completed</span>
<?php } else { ?>
<span class="text text-danger">Uncompleted</span>
<?php } ?>
</td>
<td>
<span type="button" class="btn btn-primary" data-toggle="modal" data-target="#exampleModal<?php echo $task->bf_task_id?>" data-whatever="@mdo">
Edit</span>
<a class="btn btn-sm btn-danger" href="<?php echo base_url('Bf_Task/Delete_Task/'.$task->bf_task_id)?>" onclick="return confirm('Are you sure want to Delete this task ??')"> Delete </a></td>
</tr>
<div class="modal fade" id="exampleModal<?php echo $task->bf_task_id?>" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Update Task Informarion</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form action="<?php echo base_url('Bf_Task/Update_task/'.$task->bf_task_id)?>" method="post">
<div class="form-group">
<label for="recipient-name" class="col-form-label">Task Tilte:</label>
<input name="task" value="<?php echo $task->bf_task_name ?>" type="text" class="form-control" id="recipient-name">
</div>
<div class="form-group">
<label for="recipient-name" class="col-form-label">Task Status:</label>
<select name="status" class="form-control">
<option value="0">Uncompleted</option>
<option value="1">Completed</option>
</select>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Update</button>
</div>
</div>
</div>
</div>
</form>
<?php } ?>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
1 Comments
good
ReplyDelete